In immediately’s fast-paced digital world, companies are always searching for revolutionary methods to offer personalized buyer experiences that stand out. AI brokers play an important position in personalizing these experiences by understanding buyer conduct and tailoring interactions in real-time. On this article, we’ll discover how AI brokers work to ship personalized buyer experiences, study the applied sciences behind them, and talk about sensible functions throughout numerous industries to assist companies improve their buyer engagement and satisfaction.
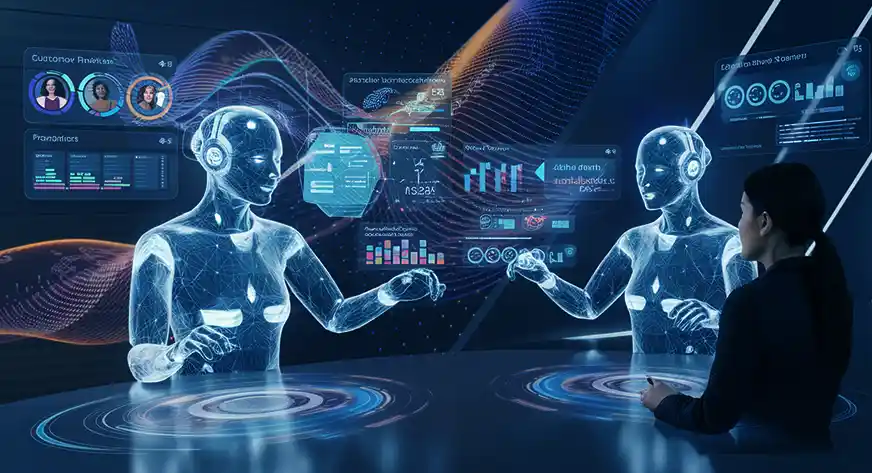
Studying Aims
- Perceive how AI brokers may be leveraged to create personalized buyer experiences by analyzing person preferences, conduct, and interactions.
- Learn to implement AI-driven options that ship customized providers and improve buyer satisfaction by personalized buyer experiences throughout numerous industries.
- Achieve insights into sensible use circumstances of AI brokers in domains like customized advertising and course of automation.
- Be taught to implement multi-agent techniques utilizing Python libraries like CrewAI and LlamaIndex.
- Develop expertise in creating and orchestrating AI brokers for real-world situations with step-by-step Python walkthroughs.
This text was printed as part of the Information Science Blogathon.
What Are AI Brokers?
AI brokers are specialised packages or fashions designed to carry out duties autonomously utilizing synthetic intelligence methods, typically mimicking human decision-making, reasoning, and studying. They work together with customers or techniques, study from knowledge, adapt to new info, and execute particular capabilities inside an outlined scope, like buyer assist, course of automation, or complicated knowledge evaluation.
In the true world, duties not often have single-step options. As an alternative, they usually contain a collection of interconnected and standalone steps to be carried out. For instance, for a query like –
“Which espresso had the best gross sales in our Manhattan based mostly retailer?” might need a single step reply.
Nevertheless, for a query like –
“Which 3 espresso varieties can be appreciated by our buyer Emily who works at Google, NYC workplace? She prefers low energy espresso and likes Lattes greater than Cappuccinos. Additionally may you ship a mail to her with a promotional marketing campaign for these 3 espresso varieties mentioning the closest location of our retailer to her workplace the place she will be able to seize these?”
A single LLM wouldn’t have the ability to deal with such a fancy question by itself and that is the place the necessity for an AI agent consisting of a number of LLMs arises.
For dealing with such complicated duties, as an alternative of prompting a single LLM, a number of LLMs may be mixed collectively performing as AI brokers to interrupt the complicated job into a number of unbiased duties.
Key Options of AI Brokers
We’ll now study key options of AI brokers intimately beneath:
- Agentic functions are constructed on a number of Language Fashions as their important framework, enabling them to supply clever, context-driven responses. These functions dynamically generate each responses and actions, adapting based mostly on person interactions. This strategy permits for extremely interactive, responsive techniques throughout numerous duties.
- Brokers are good at dealing with complicated ambiguous duties by breaking one massive job into a number of easy duties. Every of those duties may be dealt with by an unbiased agent.
- Brokers use quite a lot of specialised instruments to carry out duties, every designed with a transparent function— similar to making API requests, or conducting on-line searches.
- Human-in-the-Loop (HITL) acts as a worthwhile assist mechanism inside AI agent techniques, permitting brokers to defer to human experience when complicated conditions come up or when further context is required. This design empowers brokers to realize extra correct outcomes by combining automated intelligence with human judgment in situations that will contain nuanced decision-making, specialised information, or moral issues.
- Trendy AI brokers are multimodal and able to processing and responding to quite a lot of enter varieties, similar to textual content, photos, voice, and structured knowledge like CSV information.
Constructing Blocks of AI Brokers
AI brokers are made up of sure constructing blocks. Allow us to undergo them:
- Notion. By perceiving their surroundings, AI brokers can acquire info, detect patterns, acknowledge objects, and grasp the context during which they operate.
- Determination-making. This entails the agent selecting the simplest motion to achieve a aim, counting on the information it perceives.
- Motion. The agent carries out the chosen job, which can contain motion, sending knowledge, or different sorts of exterior actions.
- Studying. Over time, the agent enhances its skills by drawing insights from earlier interactions and suggestions, usually by machine studying strategies.
Step-by-Step Python Implementation
Allow us to contemplate a use case during which a espresso chain like Starbucks needs to construct an AI agent for drafting and mailing customized promotional campaigns recommending 3 sorts of espresso for his or her prospects based mostly on their espresso preferences. The promotional marketing campaign also needs to embrace the placement of the espresso retailer which is nearest to the shopper’s location the place the shopper can simply seize these coffees.
Step1: Putting in and Importing Required Libraries
Begin by putting in the mandatory libraries.
!pip set up llama-index-core
!pip set up llama-index-readers-file
!pip set up llama-index-embeddings-openai
!pip set up llama-index-llms-llama-api
!pip set up 'crewai[tools]'
!pip set up llama-index-llms-langchain
We’ll create the multi agent system right here utilizing CrewAI. This framework permits creation of a collaborative group of AI brokers working collectively to perform a shared goal.
import os
from crewai import Agent, Process, Crew, Course of
from crewai_tools import LlamaIndexTool
from llama_index.core import SimpleDirectoryReader, VectorStoreIndex
from llama_index.llms.openai import OpenAI
from langchain_openai import ChatOpenAI
Step2: Save Open AI Key as an Setting Variable
openai_api_key = ''
os.environ['OPENAI_API_KEY']=openai_api_key
We shall be utilizing OpenAI LLMs to question with our CSV knowledge within the subsequent steps. Therefore defining the OpenAI key right here as an surroundings variable is important right here.
Step3: Load Information utilizing LlamaIndex’s SimpleDirectoryReader
We shall be utilizing the Starbucks Information right here which has info on several types of Starbucks Espresso together with their dietary info.
reader = SimpleDirectoryReader(input_files=["starbucks.csv"])
docs = reader.load_data()
Step4: Create Question Device For Interacting With the CSV Information
#we have now used gpt-4o mannequin right here because the LLM. Different OpenAI fashions may also be used
llm = ChatOpenAI(temperature=0, mannequin="gpt-4o", max_tokens=1000)
#creates a VectorStoreIndex from an inventory of paperwork (docs)
index = VectorStoreIndex.from_documents(docs)
#The vector retailer is remodeled into a question engine.
#Setting similarity_top_k=5 limits the outcomes to the highest 5 paperwork which might be most much like the question,
#llm specifies that the LLM ought to be used to course of and refine the question outcomes
query_engine = index.as_query_engine(similarity_top_k=5, llm=llm)
query_tool = LlamaIndexTool.from_query_engine(
query_engine,
title="Espresso Promo Marketing campaign",
description="Use this device to lookup the Starbucks Espresso Dataset",
)
Step5: Creating the Crew Consisting of A number of Brokers
def create_crew(style):
#Agent For Selecting 3 Forms of Espresso Based mostly on Buyer Preferences
researcher = Agent(
position="Espresso Chooser",
aim="Select Starbucks Espresso based mostly on buyer preferences",
backstory="""You're employed at Starbucks.
Your aim is to suggest 3 Forms of Espresso FROM THE GIVEN DATA ONLY based mostly on a given buyer's life-style tastes %s. DO NOT USE THE WEB to suggest the espresso varieties."""%(style),
verbose=True,
allow_delegation=False,
instruments=[query_tool],
)
#Agent For Drafting Promotional Marketing campaign based mostly on Chosen Espresso
author = Agent(
position="Product Content material Specialist",
aim="""Craft a Promotional Marketing campaign that may mailed to buyer based mostly on the three Forms of the Espresso instructed by the earlier agent.Additionally GIVE ACCURATE Starbucks Location within the given location within the question %s utilizing 'web_search_tool' from the WEB the place the shopper can take pleasure in these coffees within the writeup"""%(style),
backstory="""You're a famend Content material Specialist, recognized for writing to prospects for promotional campaigns""",
verbose=True,
allow_delegation=False)
#Process For Selecting 3 Forms of Espresso Based mostly on Buyer Preferences
task1 = Process(
description="""Advocate 3 Forms of Espresso FROM THE GIVEN DATA ONLY based mostly on a given buyer's life-style tastes %s. DO NOT USE THE WEB to suggest the espresso varieties."""%(style),
expected_output="Listing of three Forms of Espresso",
agent=researcher,
)
#Process For Drafting Promotional Marketing campaign based mostly on Chosen Espresso
task2 = Process(
description="""Utilizing ONLY the insights supplied, develop a Promotional Marketing campaign that may mailed to buyer based mostly on 3 Forms of the Espresso instructed by the earlier agent.
Additionally GIVE ACCURATE Starbucks Location within the given location within the question %s utilizing 'web_search_tool' from the WEB the place the shopper can take pleasure in these coffees within the writeup. Your writing ought to be correct and to the purpose. Make it respectful and buyer pleasant"""%(style),
expected_output="Full Response to buyer on how one can resolve the difficulty .",
agent=author
)
#Outline the crew based mostly on the outlined brokers and duties
crew = Crew(
brokers=[researcher,writer],
duties=[task1,task2],
verbose=True, # You'll be able to set it to 1 or 2 to totally different logging ranges
)
outcome = crew.kickoff()
return outcome
Within the above code, we have now arrange a two-agent system the place:
- One agent recommends three sorts of Starbucks espresso based mostly on buyer preferences.
- The second agent drafts a promotional marketing campaign round these coffees, together with location info from the online.
The duties are coordinated inside a Crew, and the method kicks off with the crew.kickoff() operate, returning the ultimate results of the duty execution.
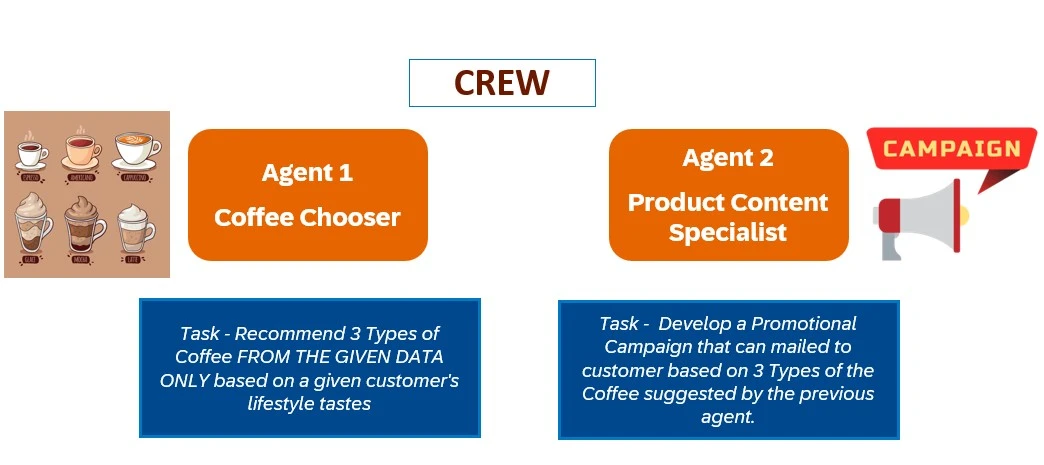
Step6: Checking Output For a Pattern Buyer
textual content = create_crew("Emily is from Gurgaon, India and likes Excessive calorie coffees and prefers Latte greater than Cappuccino.")
print(textual content)
As we will see, we need to verify the output of the MultiAgent system we created for a pattern buyer ‘Emily’ who stays in Gurgaon, India and and likes Excessive calorie coffees and prefers Latte greater than Cappuccino.
# Agent: Espresso Chooser
## Process: Advocate 3 Forms of Espresso FROM THE GIVEN DATA ONLY based mostly on a given buyer's life-style tastes Title - Emily, Location - Gurgaon, Tastes - likes Excessive calorie coffees and prefers Latte greater than Cappuccino.. DO NOT USE THE WEB to suggest the espresso varieties.
# Agent: Espresso Chooser
## Thought: I would like to collect espresso choices that match Emily's preferences for top calorie coffees, with a choice for lattes over cappuccinos.
## Utilizing device: Espresso Promo Marketing campaign
## Device Enter:
"{"question": "excessive calorie lattes"}"
## Device Output:
The high-calorie lattes embrace the Caffè Latte with 2% Milk, which has 240 energy, and the Caffè Latte with Soymilk, which has 190 energy.
# Agent: Espresso Chooser
## Thought: Thought: I must carry out one other search to search out extra latte choices which might be excessive in energy to fulfill Emily's preferences.
## Utilizing device: Espresso Promo Marketing campaign
## Device Enter:
"{"question": "excessive calorie coffees with latte base"}"
## Device Output:
Excessive-calorie espresso choices with a latte base embrace:
1. Vanilla Latte (Or Different Flavoured Latte) with 2% Milk, Venti dimension, which has 320 energy.
2. Vanilla Latte (Or Different Flavoured Latte) with Soymilk, which has 270 energy.
# Agent: Espresso Chooser
## Ultimate Reply:
1. Caffè Latte with 2% Milk (240 energy)
2. Vanilla Latte with 2% Milk, Venti dimension (320 energy)
3. Caffè Latte with Soymilk (190 energy)
# Agent: Product Content material Specialist
## Process: Utilizing ONLY the insights supplied, develop a Promotional Marketing campaign that may mailed to buyer based mostly on 3 Forms of the Espresso instructed by the earlier agent.
Additionally GIVE ACCURATE Starbucks Location within the given location within the question Title - Emily, Location - Gurgaon, Tastes - likes Excessive calorie coffees and prefers Latte greater than Cappuccino. utilizing 'web_search_tool' from the WEB the place the shopper can take pleasure in these coffees within the writeup. Your writing ought to be correct and to the purpose. Make it respectful and buyer pleasant
# Agent: Product Content material Specialist
## Ultimate Reply:
Expensive Emily,
We're thrilled to attach with you and share an thrilling lineup of coffees tailor-made to your love for high-calorie drinks and choice for lattes! Deal with your self to our fastidiously crafted choices, excellent on your style buds.
1. **Caffè Latte with 2% Milk (240 energy)**: This basic choice combines wealthy espresso with steamed 2% milk for a splendidly creamy expertise. Benefit from the excellent steadiness of flavors whereas indulging in these energy!
2. **Vanilla Latte with 2% Milk, Venti Measurement (320 energy)**: Elevate your day with our pleasant Vanilla Latte! The infusion of vanilla syrup provides a candy contact to the strong espresso and creamy milk, making it a luscious alternative that checks all of your containers.
3. **Caffè Latte with Soymilk (190 energy)**: For a barely lighter but satisfying variant, attempt our Caffè Latte with soymilk. This drink maintains the creamy goodness whereas being a tad decrease on energy, excellent for a mid-day refreshment!
To expertise these luxurious lattes, go to us at:
**Starbucks Location**:
Starbucks at **Galleria Market, DLF Section 4, Gurgaon**
Tackle: Store No. 32, Floor Ground, Galleria Market, DLF Section 4, Gurugram, Haryana 122002, India.
We will not wait so that you can dive into the pleasant world of our lattes! Cease by and savor these fantastically crafted drinks that can absolutely brighten your day.
We're right here to make your espresso moments memorable.
Heat regards,
[Your Name]
Product Content material Specialist
Starbucks
Ultimate Output Evaluation
- As we will see within the last output, three espresso varieties are really useful based mostly on the shopper’s preferences – Caffè Latte with 2% Milk (240 energy), Vanilla Latte with 2% Milk, Venti Measurement (320 energy), Caffè Latte with Soymilk (190 energy).
- All the suggestions are excessive calorie drinks and Lattes particularly for the reason that question talked about that Emily prefers excessive calorie coffees and Lattes.
- Additionally, we will see within the drafted promotional marketing campaign {that a} Starbucks location in Gurgaon has been precisely talked about.
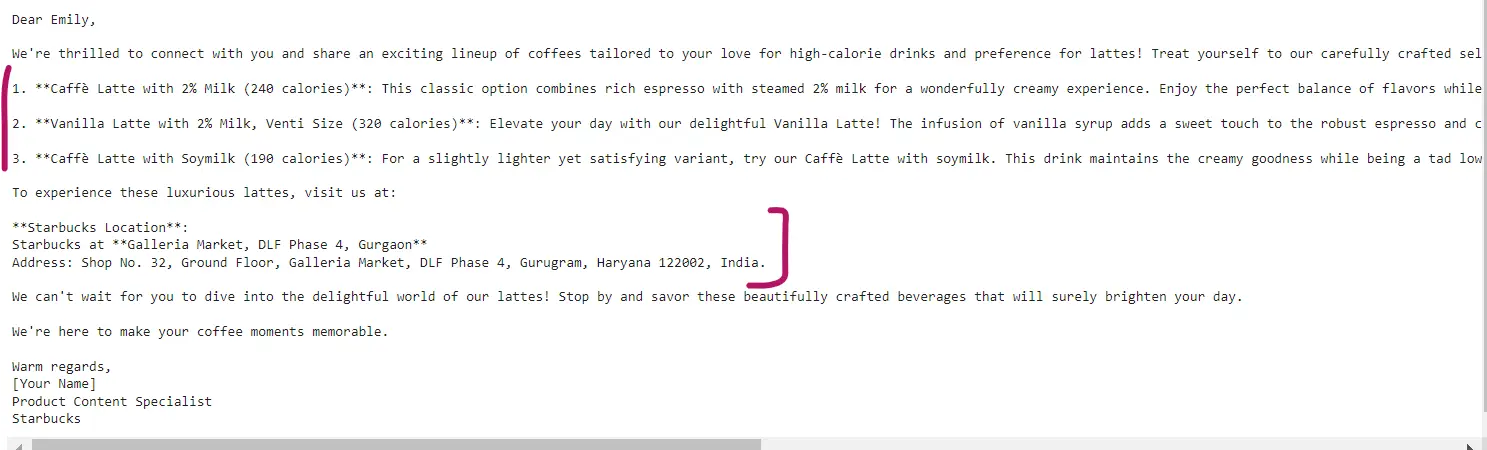
Step7: Agent For Automating the Technique of Mailing Campaigns to Clients
from langchain.brokers.agent_toolkits import GmailToolkit
from langchain import OpenAI
from langchain.brokers import initialize_agent, AgentType
toolkit = GmailToolkit()
llm = OpenAI(temperature=0, max_tokens=1000)
agent = initialize_agent(
instruments=toolkit.get_tools(),
llm=llm,
agent=AgentType.STRUCTURED_CHAT_ZERO_SHOT_REACT_DESCRIPTION,
)
print(agent.run("Ship a mail to [email protected] with the next textual content %s"%(textual content)))
We’ve utilized Langchain’s GmailToolKit (Reference Doc) right here to ship mail to our buyer, Emily’s mail id ([email protected]) with the beforehand generated textual content. To make use of Langchain’s GmailToolKit, you’ll need to arrange your credentials defined within the Gmail API docs. When you’ve downloaded the credentials.json file, you can begin utilizing the Gmail API.
Course of for Fetching the credentials.json
- An software that authenticates to Google (for instance, in our case LangChain’s GmailToolKit) utilizing OAuth 2.0 should present two objects in GCP – OAuth consent display and OAuth Shopper ID.
- To supply the OAuth consent display and OAuth consumer ID, it’s essential to create a Google Cloud Platform (GCP) venture first on the developer console.
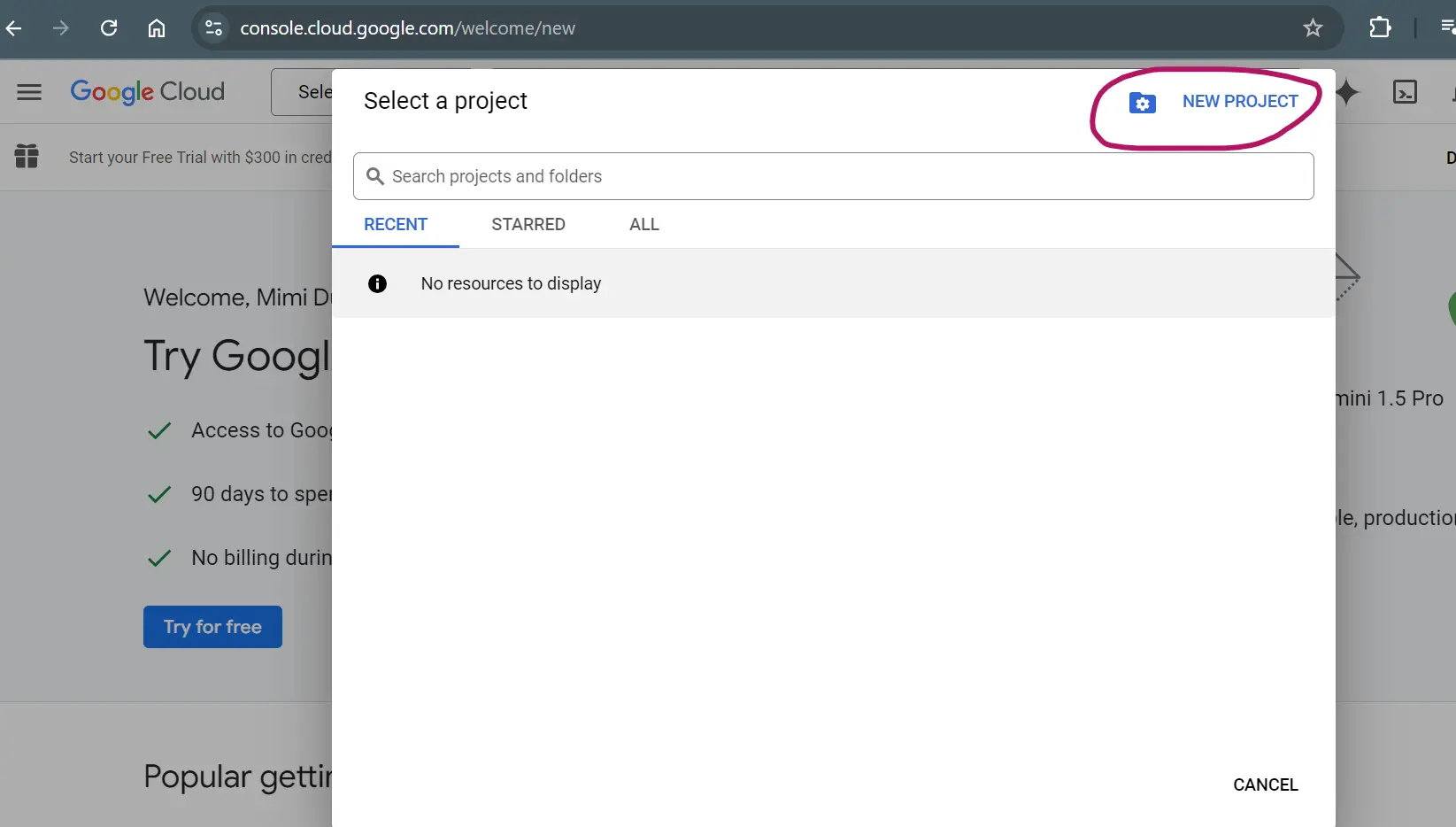
- Additionally go to “Gmail API” on the developer console and click on on “Allow”.
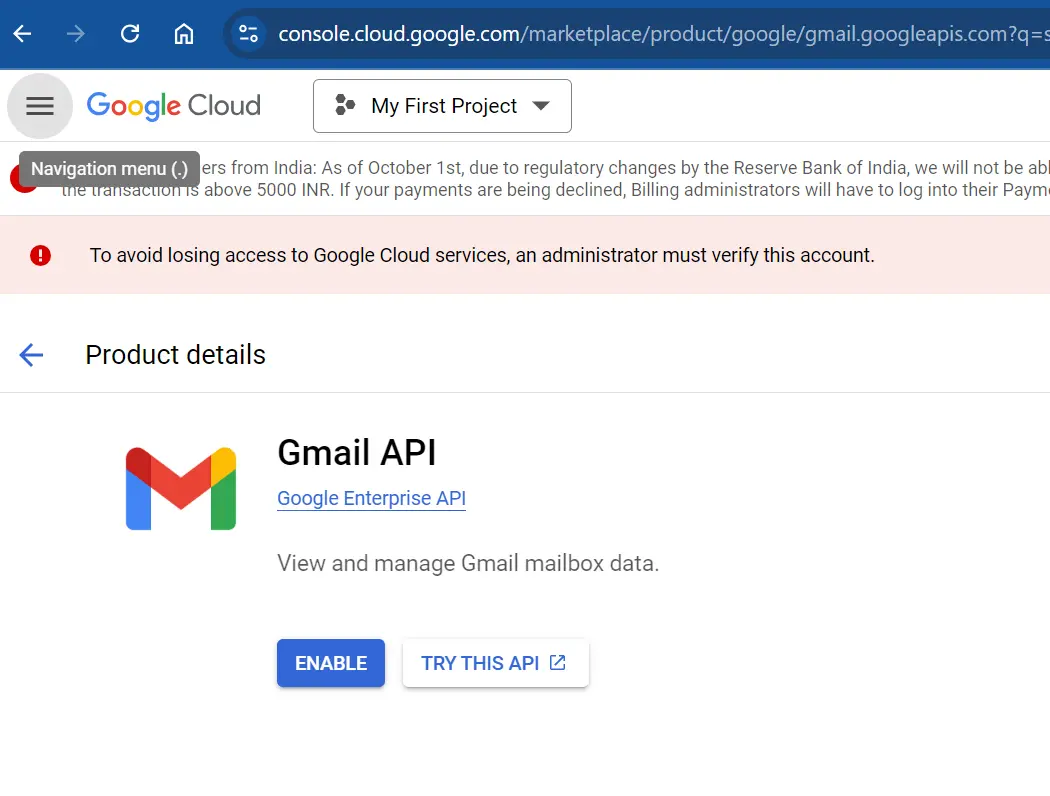
Configuring the OAuth Consent Display screen
- To open the OAuth consent display creator, choose APIs & Companies » OAuth consent display in your GCP venture.
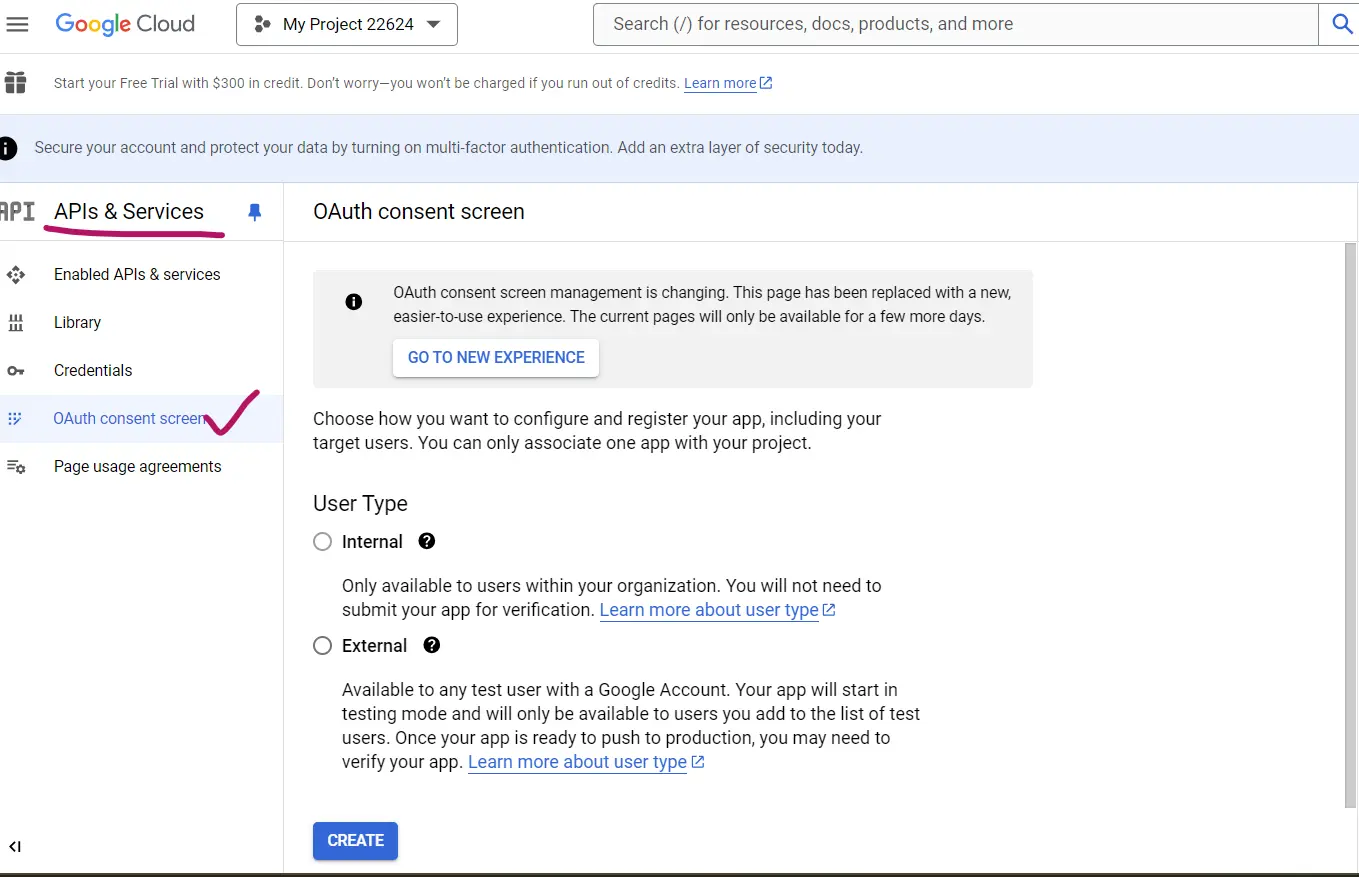
- Choose the person kind as Exterior person kind.
You’ll be able to choose the Inside person kind provided that the GCP venture belongs to a company and the connector customers are members of the identical group.
The Exterior person kind causes the authentication to run out in seven days. Should you select this sort, it is advisable to renew authentication weekly.
Present the next info:
- App title
- Consumer assist e mail: your e mail tackle
- Developer contact info: your e mail tackle
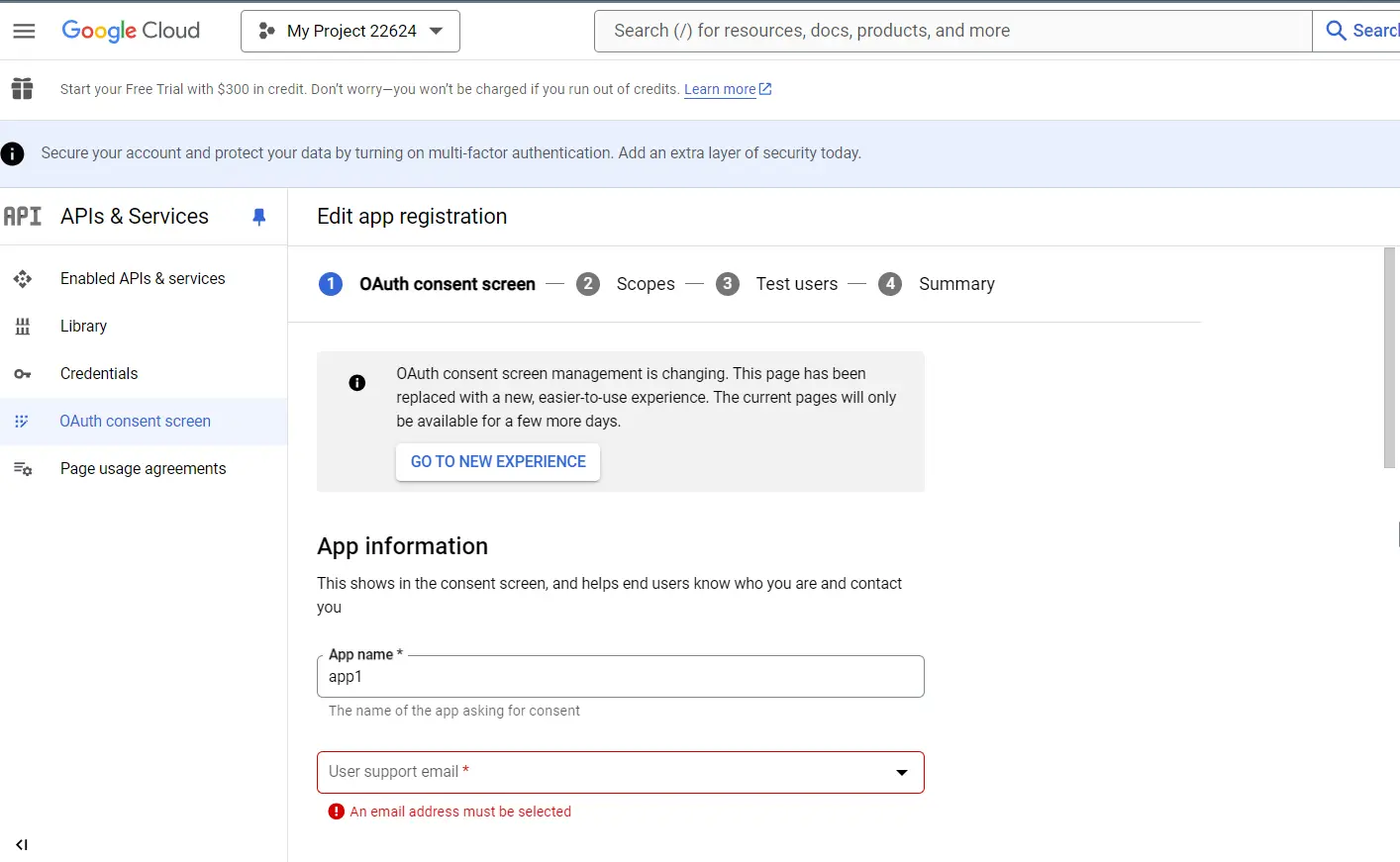
- Choose Save and proceed.
For Exterior person kind:
- Choose Take a look at customers » Add customers.
- Enter the e-mail addresses of customers which might be allowed to make use of the connector.
- Choose Add.
To complete configuration, choose Save and proceed » Again to dashboard.
Configuring the OAuth consumer ID
The next process describes how one can configure the OAuth Shopper ID:
- To open the OAuth consent display creator, choose APIs & Companies » Credentials in your GCP venture.
- Choose Create credentials » OAuth consumer ID.
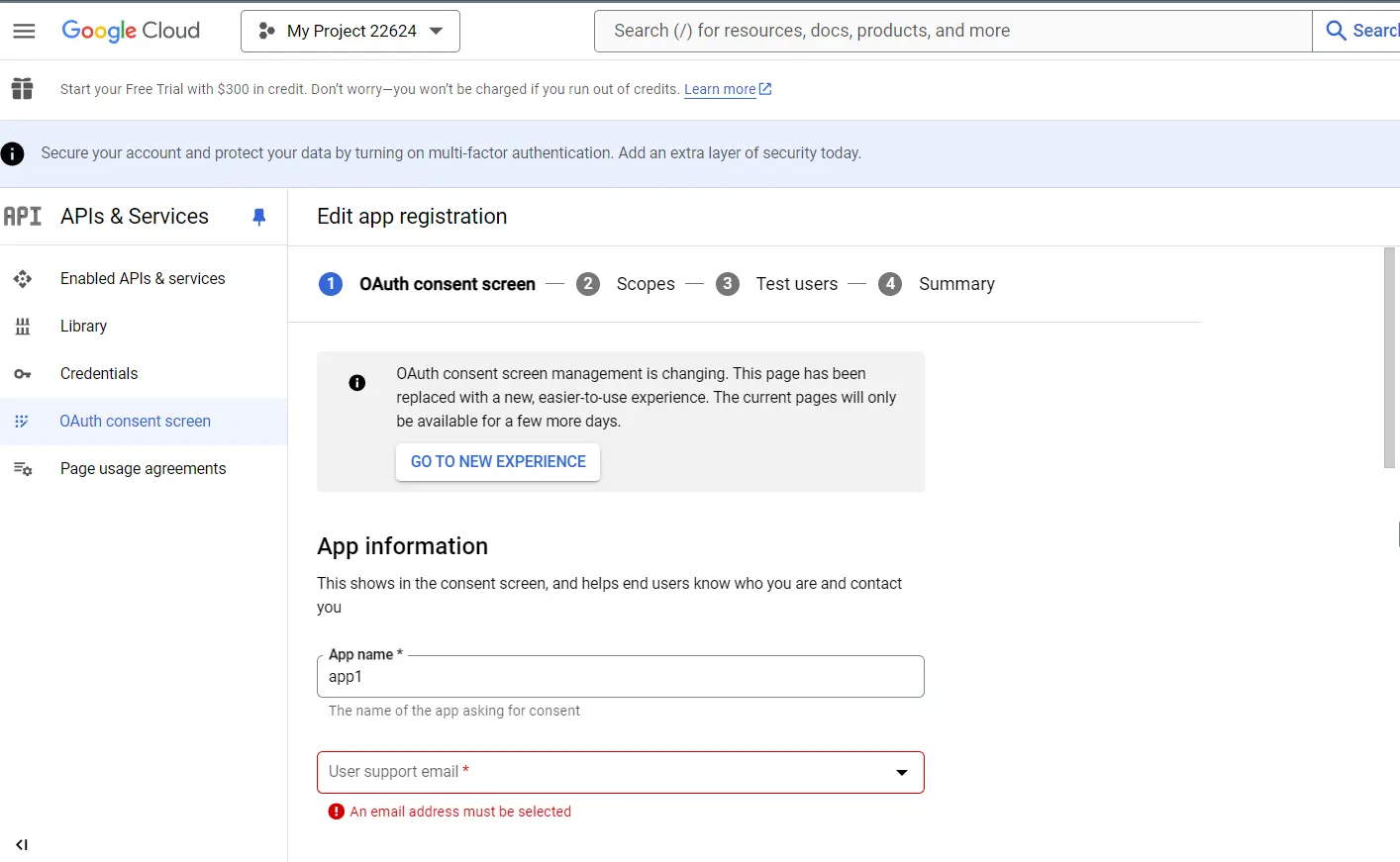
- Within the Software kind dropdown listing, choose Desktop App.
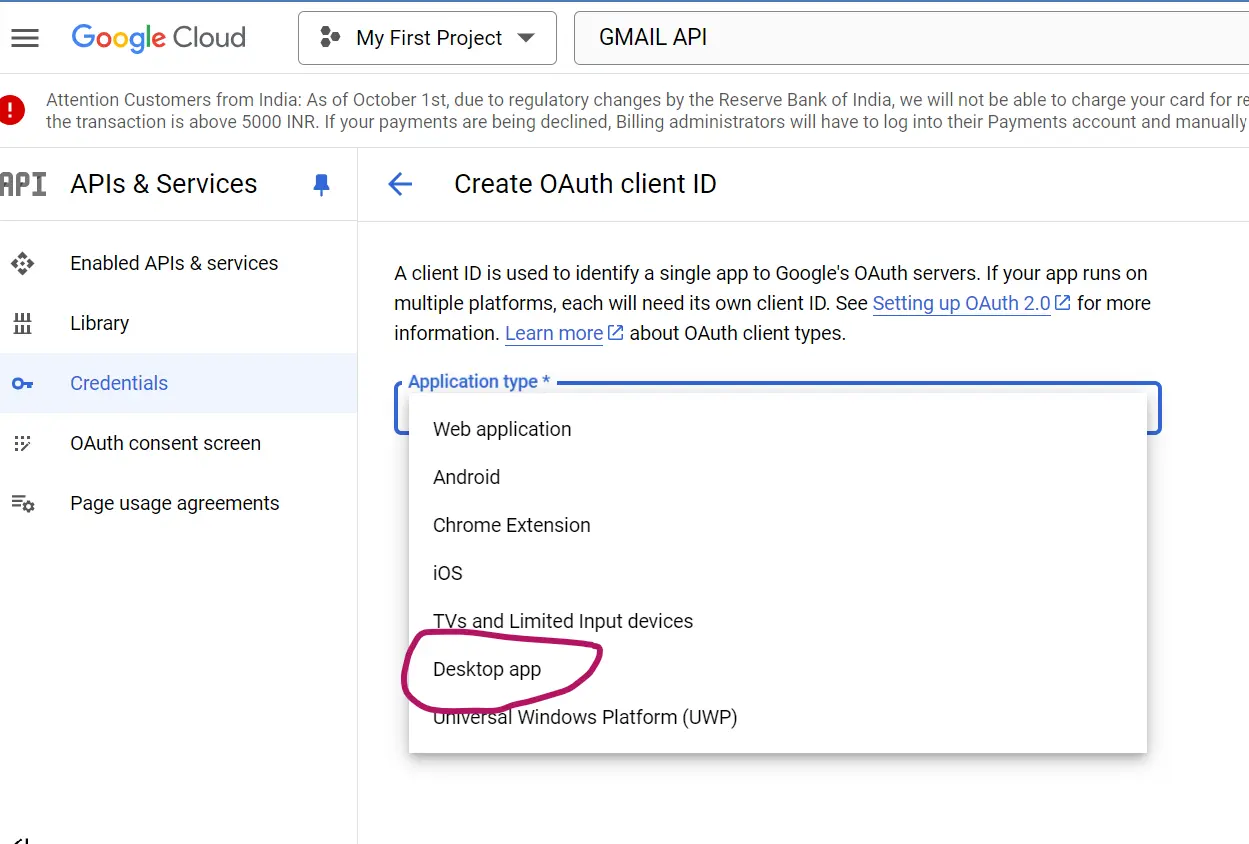
- Within the Title field, enter the specified title
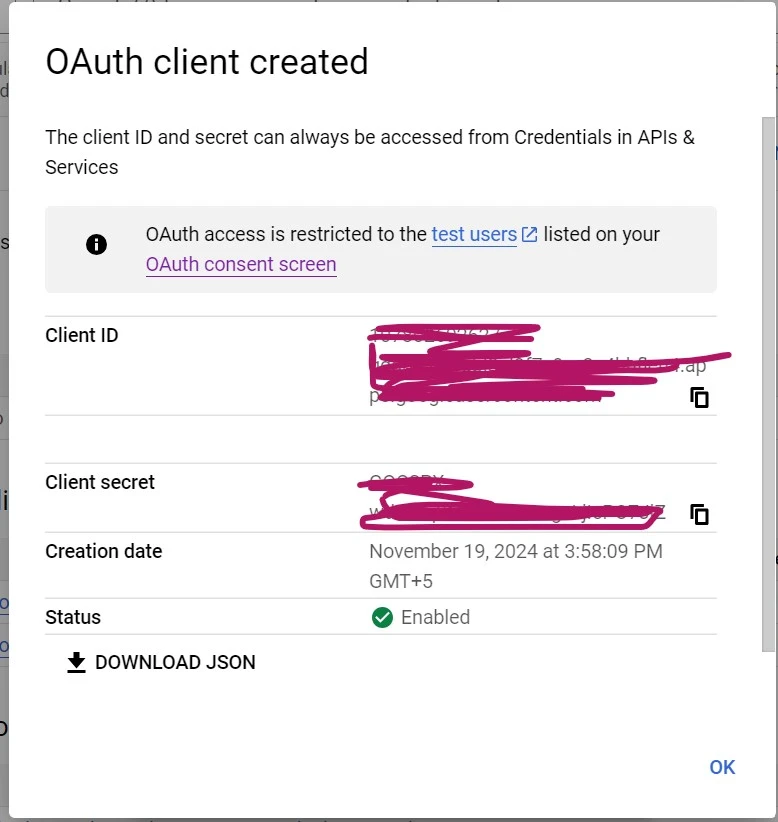
Submit clicking on “Create”, the above window will come out that can give the Shopper ID and Shopper Secret. This may be saved in a JSON format utilizing the “DOWNLOAD JSON” choice. Submit downloading, we will save this JSON file in our venture folder with the title “credentials.json”. After getting this credentials.json within the native folder, solely you then would have the ability to use the GmailToolKit.
Pattern Mail Output on Promotional Marketing campaign (despatched utilizing the above code on AI Agent)
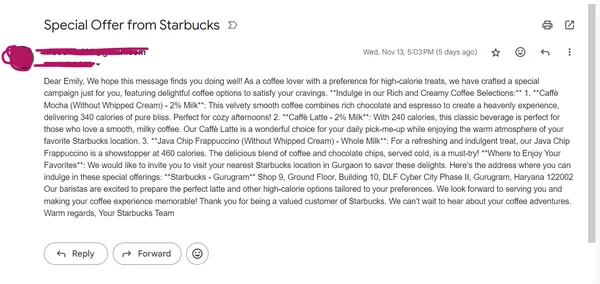
With this, we will absolutely automate the method of making and sending customized promotional campaigns, making an allowance for prospects’ distinctive life, preferences, and geographical areas. By analyzing knowledge from buyer interactions, previous purchases, and demographic info, this multi agent AI system can craft tailor-made suggestions which might be extremely related to every particular person. This stage of personalization ensures that advertising content material resonates with prospects, enhancing the possibilities of engagement and conversion.
For advertising groups managing massive buyer bases, AI brokers eradicate the complexity of focusing on people based mostly on their preferences, permitting for environment friendly and scalable advertising efforts. Consequently, companies can ship simpler campaigns whereas saving time and assets.
Challenges of AI brokers
We’ll now talk about the challenges of AI brokers beneath:
- Restricted context. LLM brokers are able to processing solely a small quantity of knowledge without delay, which may end up in them forgetting important particulars from earlier elements of a dialog or overlooking vital directions.
- Instability in Outputs. Inconsistent outcomes happen when LLM brokers, relying on pure language to interact with instruments and databases, generate unreliable outcomes. Formatting errors or failure to comply with directions precisely can occur, resulting in errors within the execution of duties.
- Nature of Prompts. The operation of LLM brokers is determined by prompts, which should be extremely correct. A minor modification can result in main errors, making the method of crafting and refining these prompts each delicate and important.
- Useful resource Necessities. Working LLM brokers requires vital assets. The necessity to course of massive volumes of information quickly can incur excessive prices and, if not correctly managed, could end in slower efficiency.
Conclusion
AI brokers are superior packages designed to carry out duties autonomously by leveraging AI methods to imitate human decision-making and studying. They excel at managing complicated and ambiguous duties by breaking them into easier subtasks. Trendy AI brokers are multimodal, able to processing numerous enter varieties similar to textual content, photos, and voice. The core constructing blocks of an AI agent embrace notion, decision-making, motion, and studying capabilities. Nevertheless, these techniques face challenges similar to restricted context processing, output instability, immediate sensitivity, and excessive useful resource calls for.
As an illustration, a multi-agent system was developed in Python for Starbucks to create customized espresso suggestions and promotional campaigns. This method utilized a number of brokers: one targeted on deciding on coffees based mostly on buyer preferences, whereas one other dealt with the creation of focused promotional campaigns. This revolutionary strategy allowed for environment friendly, scalable advertising with extremely customized content material.
Key Takeaways
- AI brokers assist create personalized buyer experiences by analyzing person knowledge and preferences to ship customized interactions that improve satisfaction and engagement.
- Leveraging AI brokers for complicated duties permits companies to create personalized buyer experiences, offering tailor-made options that anticipate wants and enhance total service high quality.
- Device integration and human-in-the-loop approaches improve AI brokers’ accuracy and capabilities.
- Multimodal options enable AI brokers to work seamlessly with numerous knowledge varieties and codecs.
- Python frameworks like LlamaIndex and CrewAI simplify constructing multi-agent techniques.
- Actual-world use circumstances show AI brokers’ potential in customized advertising and buyer engagement.
Ceaselessly Requested Questions
A. AI brokers are specialised packages that carry out duties autonomously utilizing AI methods, mimicking human decision-making and studying. Not like conventional AI fashions that always concentrate on single duties, AI brokers can deal with complicated, multi-step processes by interacting with customers or techniques and adapting to new info.
A. AI brokers encounter difficulties similar to restricted context processing, output instability, immediate sensitivity, and excessive useful resource necessities. These challenges can impression their capability to ship constant and correct leads to sure situations.
A. AI brokers use specialised instruments like API requests, on-line searches, and different task-specific utilities to carry out actions. These instruments have clear functions, guaranteeing environment friendly job completion.
A. AI brokers are obligatory for complicated duties as a result of real-world issues typically contain interconnected steps that can not be solved in a single go. AI brokers, particularly multi-agent techniques, break these duties into smaller, manageable subtasks, every dealt with independently.
The media proven on this article just isn’t owned by Analytics Vidhya and is used on the Creator’s discretion.